Python program to find factorial of a number using Recursion
Subject: Python Programming
Contributed By: Nunugoppula Ajay
Created At: February 24, 2025
Question:
Write a Python program to find factorial of a number using Recursion.
Explanation Video:
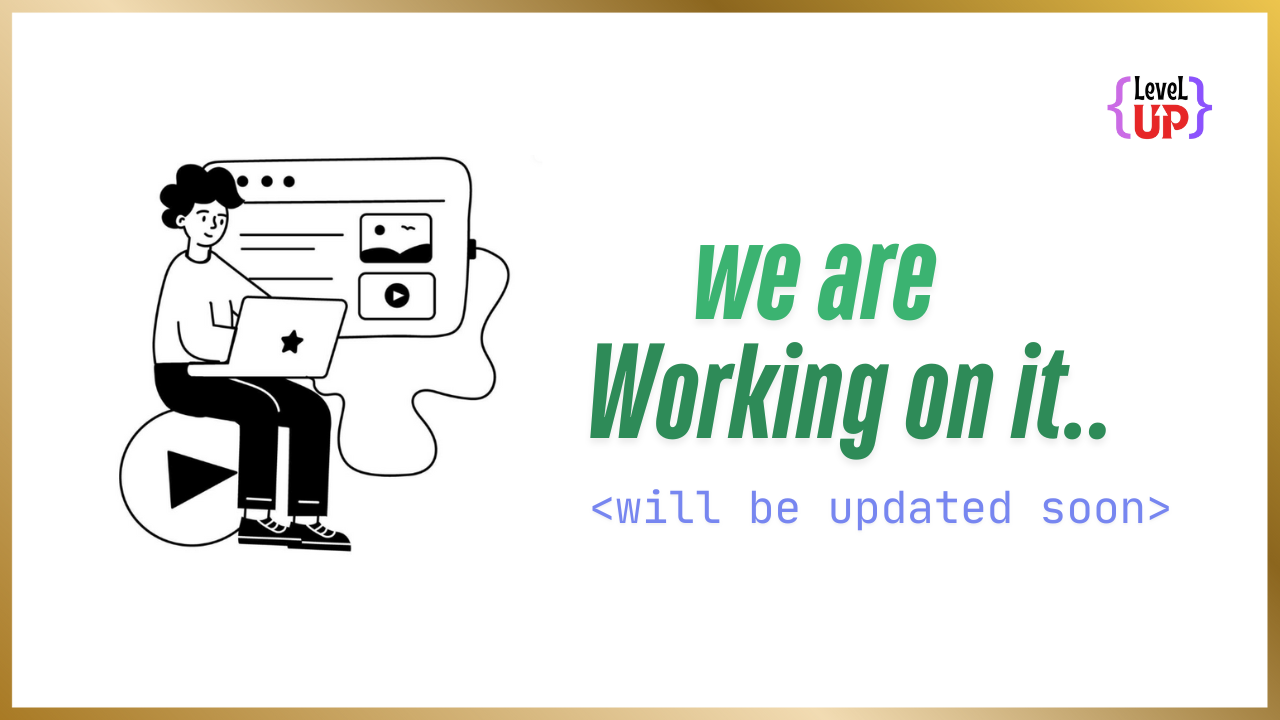
Explanation:
Factorial of a number is the cumulative product of all positive integers from 1 to the that number.
Example:
Factorial of 5 = 1x2x3x4x5 = 120
Here we used recursion, whereas implementing using for loop is very simple.
Using recursion, we need to be mindful of two things:
Base condition: The condition at which the recursive function terminates.
if num<=1: return 1
Main recursive call logic.
return num*fact(num-1)
What is Recursion?
The process of invoking the function itself within the same function, which typically terminates once the base condition is executed.
Source Code:
def fact(num):
if num<=1:
return 1
else:
return num*fact(num-1)
num=int(input("Enter a Number: "))
print("Factorial of",num,"is",fact(num))
Input:
Enter a Number: 5
Output:
Factorial of 5 is 120