Python program to check weather the given number is prime or not
Subject: Python Programming
Contributed By: Nunugoppula Ajay
Created At: February 25, 2025
Question:
Write a Python program to check weather the given number is prime or not.
Explanation Video:
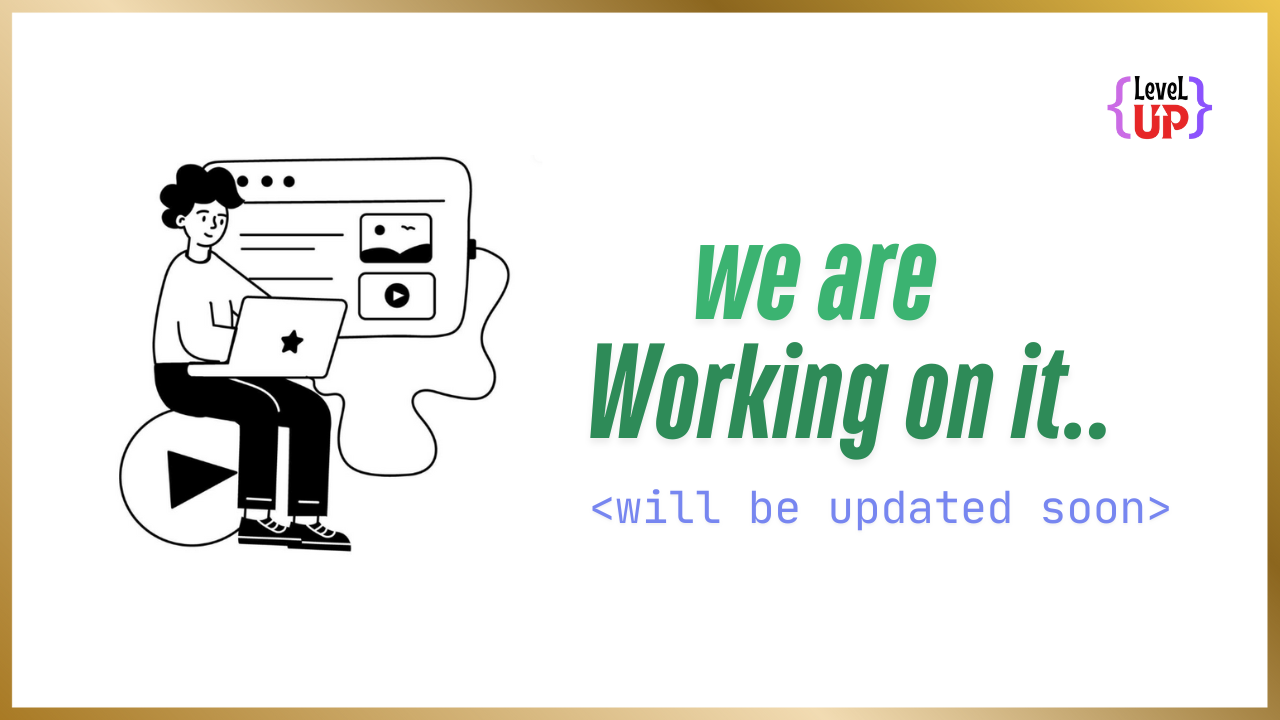
Explanation:
Source Code:
# Program to check if a number is prime or not
# Input: Get a number from the user
num = int(input("Enter a number: "))
# Initialize a counter to keep track of factors
count = 0
# Check for factors from 2 to num-1
for i in range(2, num):
if num % i == 0:
# If a factor is found, set count to 1 and break the loop
count = 1
break
# Check the count to determine if the number is prime
if count == 0 and num > 1:
print(num, "is a prime number")
else:
print(num, "is not a prime number")
Input:
Testcase-1:
Enter a number: 17
Testcase-2:
Enter a number: 24
Testcase-3:
Enter a number: 2
Testcase-4:
Enter a number: 1
Output:
Testcase-1:
17 is a prime number
Testcase-2:
24 is not a prime number
Testcase-3:
2 is a prime number
Testcase-4:
1 is not a prime number