Python program that prints prime numbers in between two numbers.
Subject: Python Programming
Contributed By: Nunugoppula Ajay
Created At: February 24, 2025
Question:
Write a Python program that prints prime numbers in between two numbers.
Explanation Video:
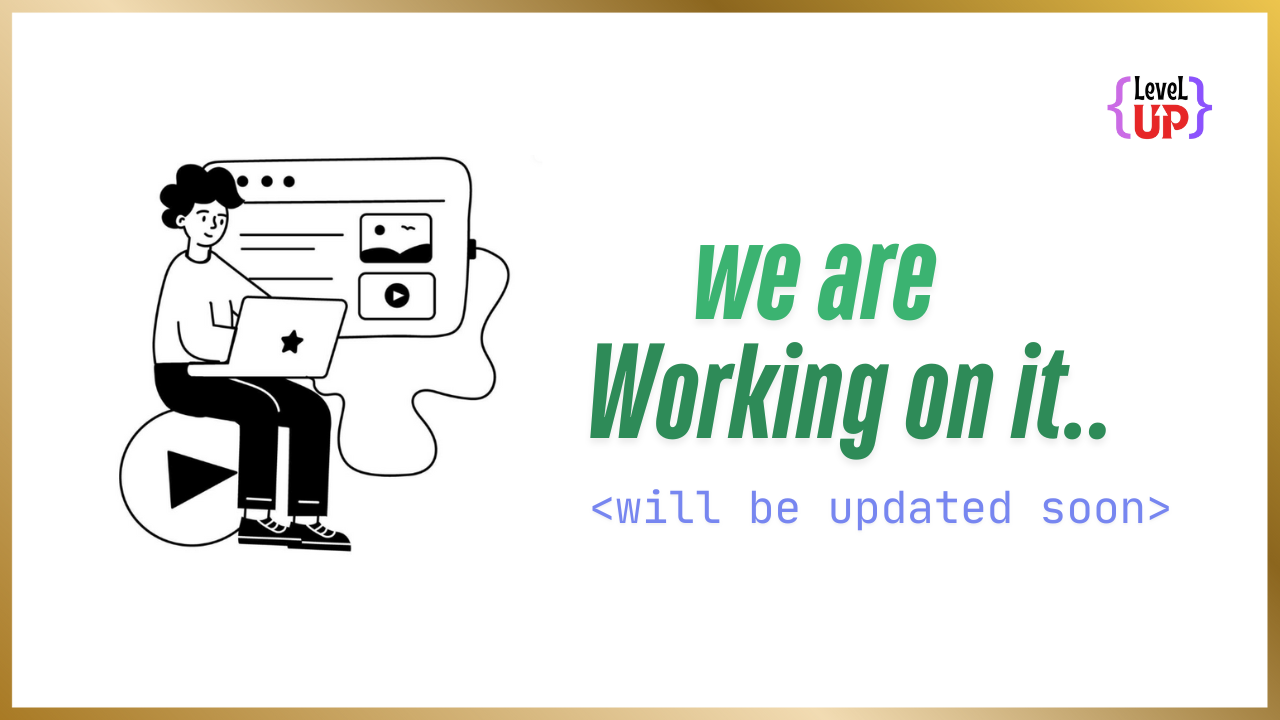
Explanation:
This is a simple way of writing a program in Python to print prime numbers between two user-given numbers.
Breakdown of code:
First, we take the user's start and end of two boundary numbers from the user using the input() function.
start=input("Enter start value: ")
end=input("Enter end value: ")
Then we use a for loop through which we iterate through numbers between two given boundaries
for num in range(start,end+1):
and then we use another for
loop factor in range(2,num):
to execute the main prime number logic, we know that the prime is nothing but the number that is only divided by itself and by 1.
After running from 2 to num, if a break statement is not executed, it will proceed to the else case beneath the inner for loop, which prints that number.
for factor in range(2,num):
if(num % factor)==0:
break
else:
print(num)
From there, execution is moved to the outer loop, which increments by 1 and again checks to see if the number is prime. If there is no factor between 2 and the current number, we break the inner loop.
start=input("Enter start value: ")
end=input("Enter end value: ")
for num in range(start,end+1):
if(num>1):
for factor in range(2,num):
if(num % factor)==0:
break
else:
print(num)
Enter start value: 50
Enter end value: 100
53
59
61
67
71
73
79
83
89
97