C Program using the I/O system calls of UNIX/LINUX operating system
Subject: OS (Operating Systems)
Contributed By: Nunugoppula Ajay
Created At: March 6, 2025
Question:
Write programs using the I/O system calls of UNIX/LINUX operating system (stat, opendir, readdir)?
Explanation Video:
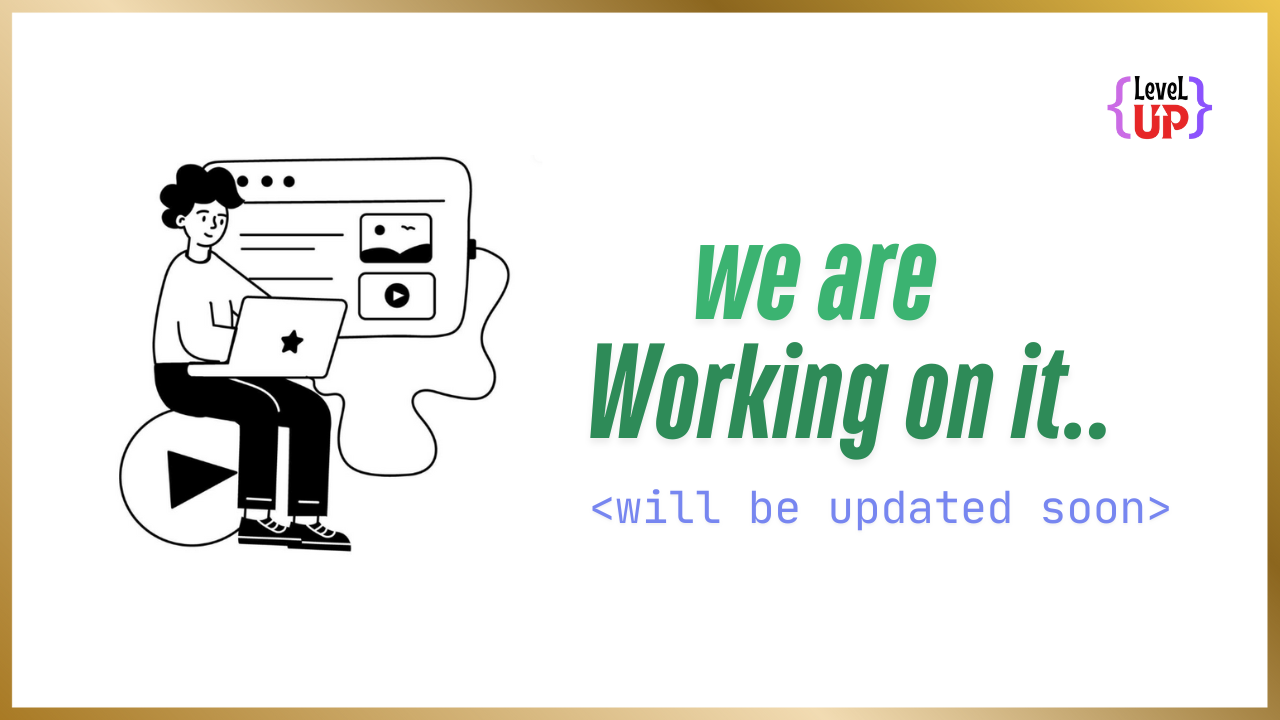
Explanation:
Source Code:
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <dirent.h>
#include <time.h>
void list_directory(const char *path) {
struct dirent *entry; // Struct for directory entries
struct stat fileStat; // Struct for file information
DIR *dir = opendir(path); // Open directory
if (dir == NULL) {
perror("Error opening directory");
exit(1);
}
printf("\nContents of directory: %s\n", path);
printf("---------------------------------------------\n");
printf("Filename\tSize (bytes)\tLast Modified\n");
printf("---------------------------------------------\n");
while ((entry = readdir(dir)) != NULL) {
char fullpath[1024];
snprintf(fullpath, sizeof(fullpath), "%s/%s", path, entry->d_name);
// Get file statistics
if (stat(fullpath, &fileStat) == -1) {
perror("Error getting file info");
continue;
}
// Print filename, size, and last modified time
printf("%-15s %-10ld %s", entry->d_name, fileStat.st_size, ctime(&fileStat.st_mtime));
}
closedir(dir); // Close directory
}
int main() {
char directory[256];
printf("Enter directory path: ");
scanf("%s", directory);
list_directory(directory);
return 0;
}
Output:
# EXAMPLE OUTPUT:
Enter directory path: .
Contents of directory: .
---------------------------------------------
Filename Size (bytes) Last Modified
---------------------------------------------
file1.txt 1024 Mon Mar 4 12:30:10 2024
script.sh 512 Sun Mar 3 18:45:30 2024
my_program 20480 Sat Mar 2 10:15:20 2024