C Program for Priority Scheduling algorithm
Subject: OS (Operating Systems)
Contributed By: Nunugoppula Ajay
Created At: March 6, 2025
Question:
Write a C Program for Priority-based Scheduling algorithm in OS.
Explanation Video:
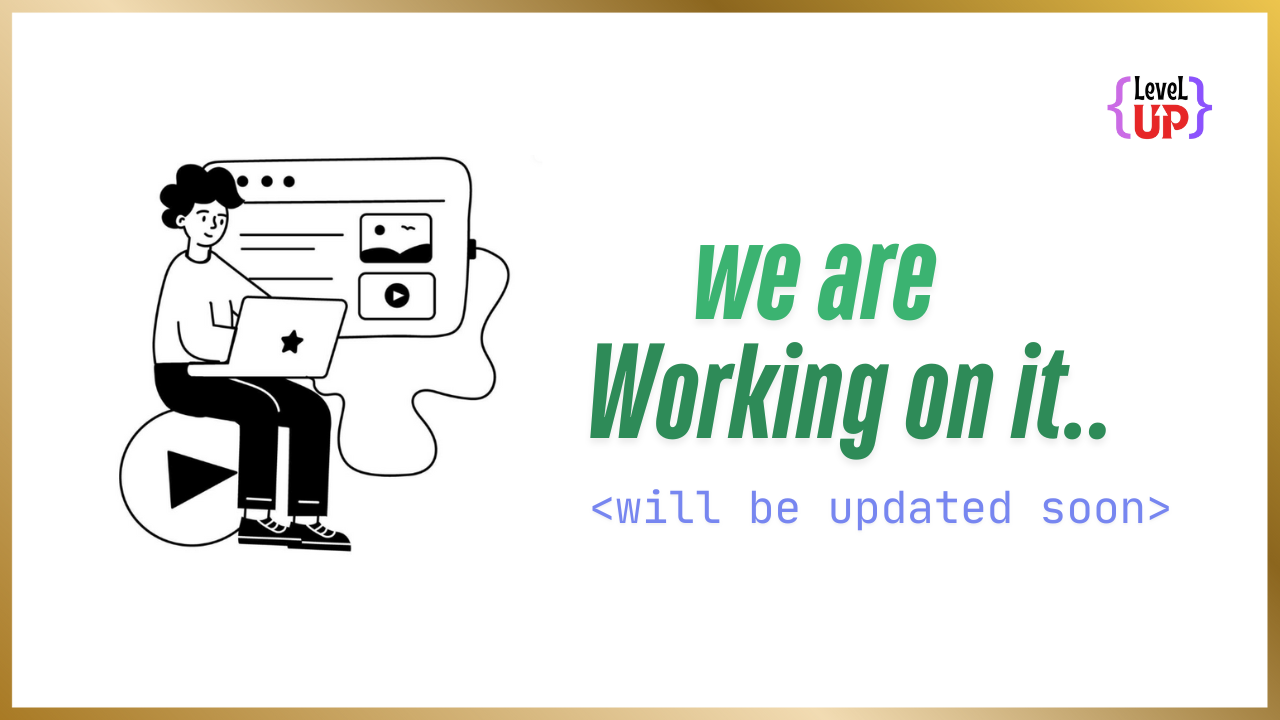
Explanation:
Source Code:
#include <stdio.h>
// Structure to store process details
struct Process {
int id; // Process ID
int bt; // Burst Time
int wt; // Waiting Time
int tat; // Turnaround Time
int priority; // Priority
};
// Function to calculate Waiting Time
void calculateWaitingTime(struct Process p[], int n) {
p[0].wt = 0; // First process has zero waiting time
for (int i = 1; i < n; i++) {
p[i].wt = p[i - 1].wt + p[i - 1].bt;
}
}
// Function to calculate Turnaround Time
void calculateTurnaroundTime(struct Process p[], int n) {
for (int i = 0; i < n; i++) {
p[i].tat = p[i].bt + p[i].wt;
}
}
// Function to sort processes by Priority (Lower value = Higher priority)
void sortByPriority(struct Process p[], int n) {
struct Process temp;
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (p[i].priority > p[j].priority) {
temp = p[i];
p[i] = p[j];
p[j] = temp;
}
}
}
}
// Function to display the process details and compute averages
void displayResults(struct Process p[], int n) {
int total_wt = 0, total_tat = 0;
printf("\nProcess\tBurst Time\tPriority\tWaiting Time\tTurnaround Time\n");
for (int i = 0; i < n; i++) {
total_wt += p[i].wt;
total_tat += p[i].tat;
printf("%d\t%d\t\t%d\t\t%d\t\t%d\n", p[i].id, p[i].bt, p[i].priority, p[i].wt, p[i].tat);
}
printf("\nAverage Waiting Time: %.2f", (float)total_wt / n);
printf("\nAverage Turnaround Time: %.2f\n", (float)total_tat / n);
}
// Main function
int main() {
int n;
printf("Enter the number of processes: ");
scanf("%d", &n);
struct Process p[n];
printf("Enter the Burst Time and Priority for each process:\n");
for (int i = 0; i < n; i++) {
p[i].id = i + 1;
printf("Process %d:\n", p[i].id);
printf("Burst Time: ");
scanf("%d", &p[i].bt);
printf("Priority: ");
scanf("%d", &p[i].priority);
}
// Sorting processes based on priority
sortByPriority(p, n);
// Calculate Waiting & Turnaround Times
calculateWaitingTime(p, n);
calculateTurnaroundTime(p, n);
// Display the final process execution details
displayResults(p, n);
return 0;
}
Input:
Enter the number of processes: 3
Enter the Burst Time and Priority for each process:
Process 1:
Burst Time: 10
Priority: 2
Process 2:
Burst Time: 5
Priority: 1
Process 3:
Burst Time: 8
Priority: 3
Output:
Process Burst Time Priority Waiting Time Turnaround Time
2 5 1 0 5
1 10 2 5 15
3 8 3 15 23
Average Waiting Time: 6.67
Average Turnaround Time: 14.33