C Program for Round Robin (RR) CPU scheduling
Subject: OS (Operating Systems)
Contributed By: Nunugoppula Ajay
Created At: March 6, 2025
Question:
Write a C Program to implement Round Robin (RR) CPU scheduling Algorithm in OS
Explanation Video:
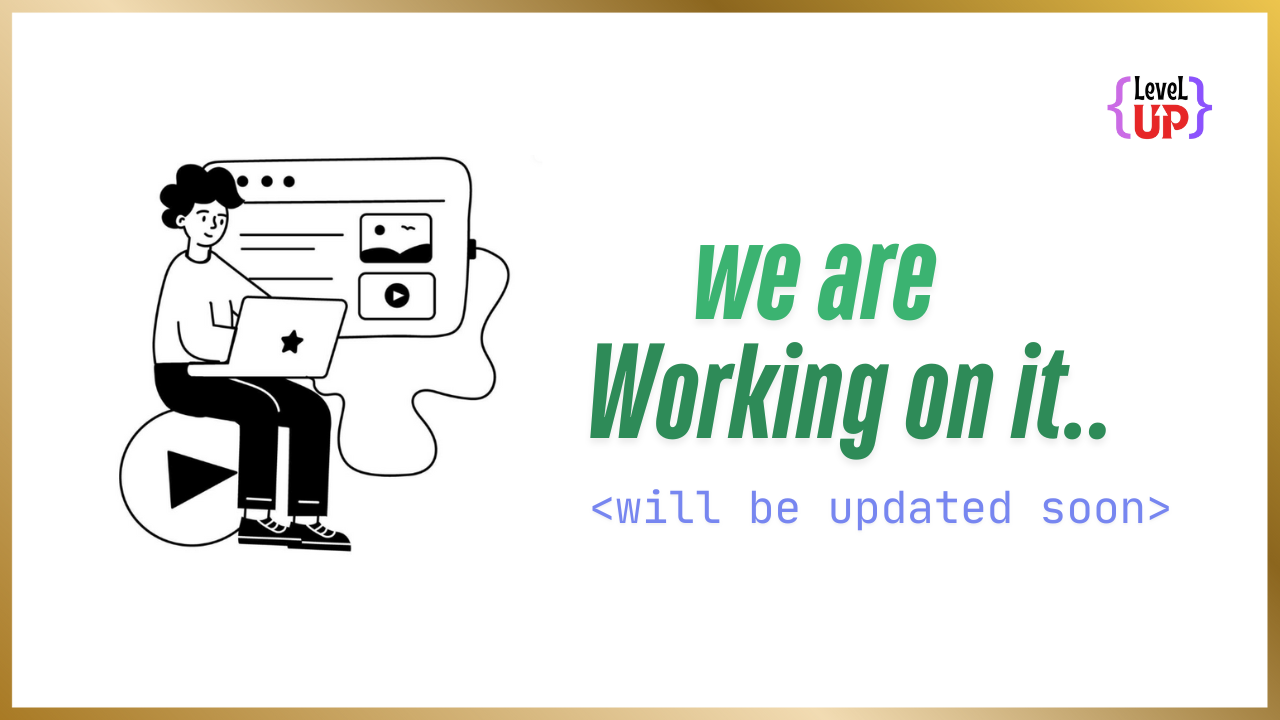
Explanation:
Source Code:
#include<stdio.h>
//Calculate the average time
void RoundRobin(int bt[],int n,int quantum)
{
int wt[n], tat[n], total_wt = 0, total_tat = 0;
// Find waiting time of all processes
//Store remaining burst time
int rem_bt[n],i;
for ( i = 0 ; i < n ; i++)
rem_bt[i] = bt[i];
int t = 0; // Current time
while (1)
{
int done = 1;
for ( i = 0 ; i < n; i++)
{
if (rem_bt[i] > 0)
{
done = 0; // pending process
if (rem_bt[i] > quantum)
{
t += quantum;
rem_bt[i] -= quantum;
}
else
{
t = t + rem_bt[i];
// Waiting time = current time- time
// used in this process
wt[i] = t - bt[i];
//process is fully executed
rem_bt[i] = 0;
}
}
}
if (done == 1)
break;
}
//Find turn around time for all processes
for (int i = 0; i < n ; i++){
tat[i] = bt[i] + wt[i];
total_wt += wt[i];
total_tat += tat[i];
}
// Calculate average waiting and turnaround times
float avg_wt = (float)total_wt / n;
float avg_tat = (float)total_tat / n;
// Display process details
printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");
printf("-------------------------------------------------\n");
for (int i = 0; i < n; i++) {
printf("%d\t%d\t\t%d\t\t%d\n", i + 1, bt[i], wt[i], tat[i]);
}
printf("\nAverage Waiting Time: %.2f\n", avg_wt);
printf("Average Turnaround Time: %.2f\n", avg_tat);
}
int main() {
int n;
// Input number of processes
printf("Enter the number of processes: ");
scanf("%d", &n);
int bt[n];
// Input burst time for each process
printf("Enter Burst Time for each process:\n");
for (int i = 0; i < n; i++) {
printf("Process %d Burst Time: ", i + 1);
scanf("%d", &bt[i]);
}
int time_quantum = 2;
// Call FCFS scheduling function
RoundRobin(bt,n,time_quantum);
return 0;
}
Input:
Enter the number of processes: 3
Enter Burst Time for each process:
Process 1 Burst Time: 5
Process 2 Burst Time: 4
Process 3 Burst Time: 6
Output:
Process Burst Time Waiting Time Turnaround Time
-------------------------------------------------
1 5 8 13
2 4 6 10
3 6 9 15
Average Waiting Time: 7.67
Average Turnaround Time: 12.67