C Program to Print Fibonacci Numbers Using Recursion
Subject: PPS (Programming for Problem Solving)
Contributed By: Sanjay
Created At: February 3, 2025
Question:
Write a C Program to Print Fibonacci Numbers Using Recursion
Explanation Video:
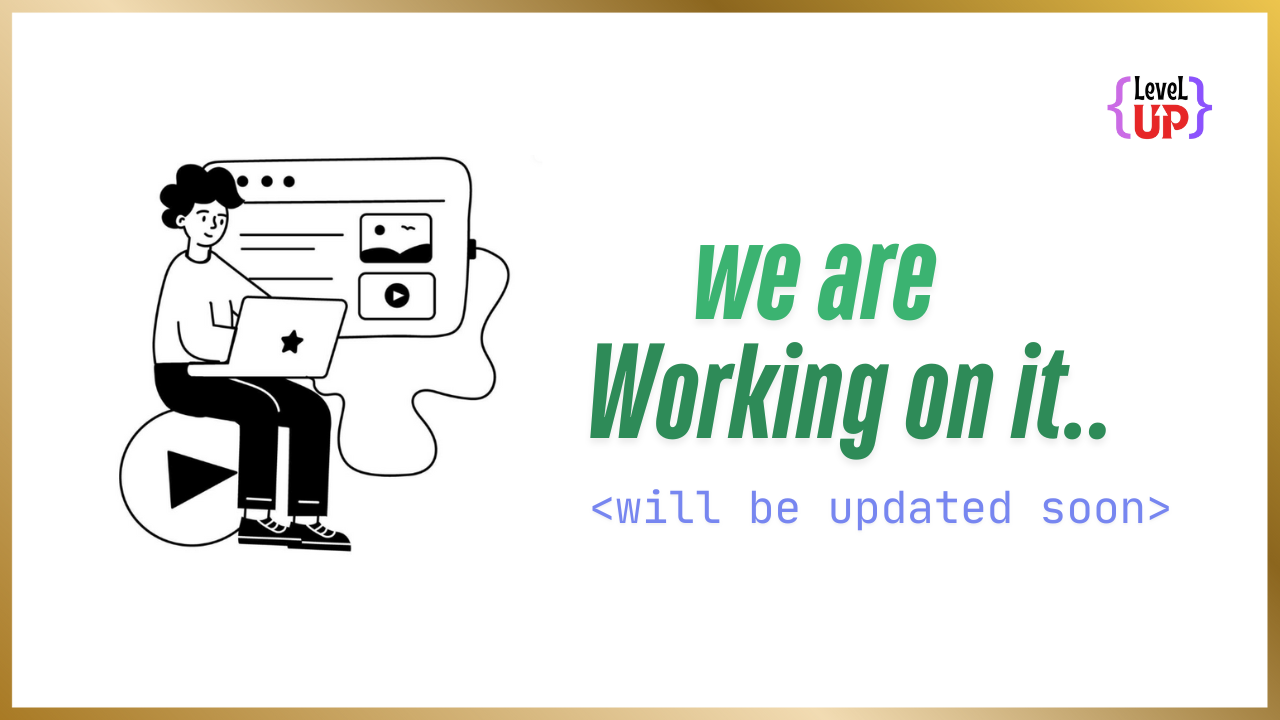
Explanation:
The Fibonacci sequence starts with 0 and 1, and each next term is the sum of the previous two terms.
We use a recursive function fibonacci(n) to compute each term.
The base case is when n == 0 or n == 1, returning 0 and 1, respectively.
Source Code:
#include <stdio.h>
int fibonacci(int n) {
if (n == 0)
return 0;
else if (n == 1)
return 1;
else
return fibonacci(n - 1) + fibonacci(n - 2);
}
int main() {
int n;
printf("Enter the number of terms: ");
scanf("%d", &n);
printf("Fibonacci sequence: ");
for (int i = 0; i < n; i++) {
printf("%d ", fibonacci(i));
}
return 0;
}
Input:
Enter the number of terms: 5
Output:
Fibonacci sequence: 0 1 1 2 3